Configure Visual Studio to Name Private Fields with Underscore
Date Published: 20 August 2019
Last updated: 13 October 2023
Most C# coding standards recommend using camelCase for local variables and _camelCase for private or internal (and I also say for protected, but that's just me) fields. Unfortunately, out of the box Visual Studio just uses camelCase for these fields, which makes typical dependency injection scenarios annoying in constructors:
// ctor
public SomeClass(ISomeService someService)
{
this.someService = someService; // annoying same name format usage
}
I much prefer this version:
// ctor
public SomeClass(ISomeService someService)
{
_someService = someService; // obvious intent; no need for 'this'
}
Fortunately, you can modify Visual Studio to do the right thing pretty easily, albeit not in a hugely discoverable manner (it's a bit buried in the options). Here's how to find what you need:
- Click on Tools in the menu.
- Click on Options.
- Click on Text Editor.
- Click on C#.
- Click on Code Style.
- Click on Naming.
- Click on Manage naming styles.
Still with me? Now you should see a Naming Style dialog. Specify the following (see image below):
- Naming Style: _fieldName
- Required Prefix: _
- Capitalization: camel Case Name
If you did it right your Sample Identifier will be _exampleIdentifier.
Naming Style dialog in Visual Studio 2019 C# Code Style - Naming - Manage naming styles.
Once you have this in place, you're almost done. Now click the green plus (+) sign to add a new specification and choose the following from the 3 dropdown lists:
- Private or Internal Fields
- _fieldName
- Suggestion
Your final list should look something like this:
Now if you use the extremely common refactoring of assigning a constructor parameter to a newly initialized field, Visual Studio will name it using the _fieldName naming rule:
Visual Studio 2019 Refactoring to Create and initialize field _fieldName.
Thanks to this StackOverflow answer which has helped me (and several clients) out with this a few times. Posting here so I'm sure to be able to find it again in the future, and to help readers like you!
You can also achieve this using a .editorconfig file. An example of this can be found in the Roslyn codebase (thanks, Rhodri!):
# Instance fields are camelCase and start with _
dotnet_naming_rule.instance_fields_should_be_camel_case.severity = suggestion
dotnet_naming_rule.instance_fields_should_be_camel_case.symbols = instance_fields
dotnet_naming_rule.instance_fields_should_be_camel_case.style = instance_field_style
dotnet_naming_symbols.instance_fields.applicable_kinds = field
dotnet_naming_style.instance_field_style.capitalization = camel_case
dotnet_naming_style.instance_field_style.required_prefix = _
Category - Browse all categories
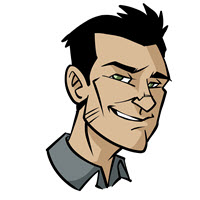
About Ardalis
Software Architect
Steve is an experienced software architect and trainer, focusing on code quality and Domain-Driven Design with .NET.