Testing Email Sending
Date Published: 17 June 2010
Recently I learned a couple of interesting things related to sending emails. One doesn't relate to .NET at all, so if you're a developer and you want to easily be able to test whether or not emails are working correctly in your application without actually sending them and/or installing a real SMTP server on your machine, pay attention. You can grab the smtp4dev application from codeplex, which will listen for SMTP emails and log them for you (and will even pop-up from the system tray to notify you when it receives them) – but it will never send them. Here's what the notification looks like:
The other interesting tidbit about emails that is specific to .NET is that the MailMessage class is IDisposable. I never realized that before, but I noticed it while reading through the Ben Watson's C# 4 How To bookI picked up at TechEd last week (which is done cookbook style and has a ton of useful info in it). Looking at the MailMessage's Dispose() method in Reflector reveals that it only really matters if you're working with AlternateViews or Attachments (this.bodyView is also of type AlternateView):
All the same, you should get in the habit of wrapping your calls to MailMessage in a using() { … }
block. Here's some sample code showing how I would typically work with MailMessage:
Bad Example (don't cut and paste and use):
string fromAddress = "nobody@somewhere.com";
string toAddress = "somebody@somewhere.com";
string subject = "Test Message from IDisposable";
string body = "This is the body.";
var myMessage = new MailMessage(fromAddress, toAddress, subject, body);
var myClient = new SmtpClient("localhost");
myClient.Send(myMessage);
And here's how that code would look once it's properly set up using IDisposable / using:
Good Example (use this one!):
string fromAddress = "nobody@somewhere.com";
string toAddress = "somebody@somewhere.com";
string subject = "Test Message from IDisposable";
string body = "This is the body.";
using(var myMessage = new MailMessage(fromAddress, toAddress, subject, body))
using(var myClient = new SmtpClient("localhost"))
{
myClient.Send(myMessage);
}
Note also that SmtpClient is also IDisposable, and that you can stack your using() statements without introducing extra { … } when you have instances where you need to work with several Disposable objects (like in this case).
Note that if you run Code Analysis in VS2010 (not sure which version you have to have – right click on a project in your solution explorer and look for Analyze Code) you will get warnings for any IDisposable classes you're using outside of using statements, like these:
[
Summary
Sometimes things you don't expect to be Disposable are. It's good to run some code analysis tools from time to time to help find things like this. Visual studio has some nice static analysis built into some versions, or you can use less expensive tools like Fx Cop or Nitriq.
Category - Browse all categories
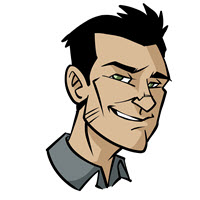
About Ardalis
Software Architect
Steve is an experienced software architect and trainer, focusing on code quality and Domain-Driven Design with .NET.