Testing Around ASP.NET Cache Features
Date Published: 08 July 2008
It’s still quite painful to try and test code that relies on ASP.NET Caching. Now, I love caching. Ask anybody. But testing around it can pretty much suck. The biggest source of pain for me at the moment is the SqlCacheDependency, which is freaking awesome in terms of functionality but freaking annoying in terms of testing around it. I have integration tests that, for some reason, occasionally fail claiming that “table X is not configured for SQL Cache dependency.” This, despite a SQL script that runs before every test and ensures that yes, in fact, those tables are configured. But even leaving integration tests out of the picture, unit testing classes that rely on logic that might touch a SqlCacheDependency is extremely difficult, because you cannot easily use Dependency Injection to mock away the dependency. And hardcoded into the SqlCacheDependency is logic that tries to talk to a real database instance (to do the aforementioned check whether the table in question is configured for the cache dependencies).
Speaking with Roy Osherove about this issue, he assured me that TypeMock handles this easily, and also pointed me toward Ivonna, which is an in-memory ASP.NET testing tool that sounds somewhat similar to the Plasma project I worked on a long while ago (except that it sounds like this one might actually go somewhere). Thus far I’ve only used Rhino Mocks for my mocking needs, but I think I’m going to have to give TypeMock a try in the near future to see how well it handles some of these issues (and if Rhino Mocks can mock SqlCacheDependency, please let me know how).
The way I handle this now is like this:
<span style="color: #0000ff">public</span> SqlCacheDependency GetSqlCacheDependency()
{
<span style="color: #0000ff">try</span>
{
System.Web.Caching.SqlCacheDependency sqlDependency =
<span style="color: #0000ff">new</span> SqlCacheDependency(LQSiteConfig.Settings.SqlCacheDatabaseName, tableDependency);
<span style="color: #0000ff">return</span> sqlDependency;
}
<span style="color: #0000ff">catch</span> (Exception ex)
{
<span style="color: #0000ff">if</span> (PersistenceLayer <span style="color: #0000ff">is</span> SqlPersistenceLayer)
{
<span style="color: #008000">// log it; otherwise ignore it</span>
}
}
<span style="color: #0000ff">return</span> <span style="color: #0000ff">null</span>;
}
Essentially this will ignore any exceptions generated by the SqlCacheDependency when I’m in a unit test, as the PersistenceLayer in that case will be mocked.
Tags - Browse all tags
Category - Browse all categories
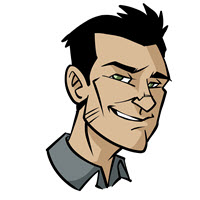
About Ardalis
Software Architect
Steve is an experienced software architect and trainer, focusing on code quality and Domain-Driven Design with .NET.